Today’s WordPress developers need to understand a variety of JavaScript methods and frameworks. This article covers the most common methods you’ll see in the wild in both older and more forward-looking WordPress code. Some examples include AJAX with jQuery, ES6, the Heartbeat API, REACT, and Gutenberg blocks.
My goal is to help you feel more comfortable opening up any JS file you come across in the WordPress world.
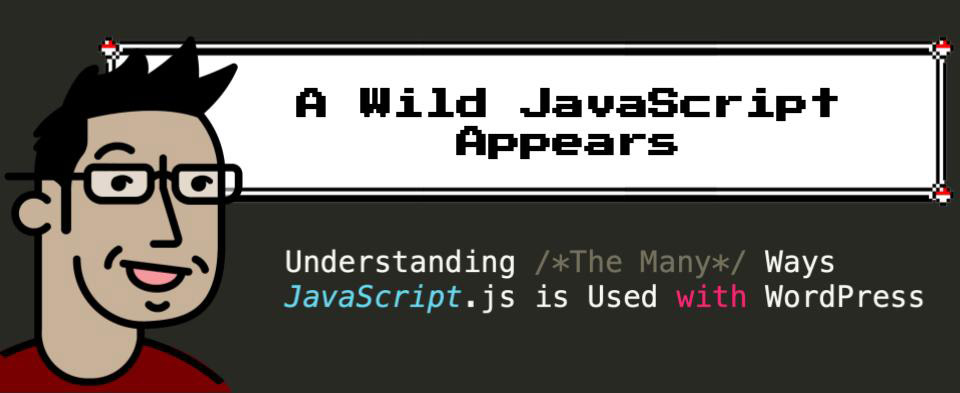
A few years ago, Matt Mullenweg asked us to learn JavaScript deeply.
I think Matt meant for PHP developers to learn JavaScript and for us to learn vanilla JavaScript in particular so we could program with it regardless of framework. Learning JavaScript deeply was important, because over the next few years, framework development continued quickly. Every year a different library, framework, or flavor of JS would become popular.
Vanilla JavaScript developed quickly as well with the base language itself gaining new features. Different browsers adopted the new features at different rates, requiring polyfill solutions, requiring compilers and package managers. I honestly don’t know how new developers pick this stuff up. The JavaScript landscape seems very confusing right now. Maybe it’s more confusing for folks like me who have been using JavaScript since the 1995.
The following sections go through 10 or so examples of JavaScript you will see in the wild with the intent of helping you to detect what kind of JavaScript, libraries, frameworks, and tools are being used.
Commonly Found Examples of JavaScript in WordPress
Vanilla JavaScript
- Photo Credit: https://www.pokemon.com/us/pokedex/Vanillite
- Code Example: https://github.com/WordPress/WordPress/blob/master/wp-includes/js/wp-list-revisions.js.
- Used For: Everything. In particular, WordPress core tries to avoid JS frameworks in any frontend code. Plugin and theme developers have been moving toward using Vanilla JS vs libraries like jQuery.
- Identifying Features: window, document, getElementById, addEventListener (most libraries and frameworks have shortcuts for these).
- Learn More: https://javascriptforwp.com/product/javascript-language-basics/, https://codex.wordpress.org/Using_Javascript
Minimized
- Photo Credit: https://www.pokemon.com/us/pokedex/Minun
- Code Example: https://github.com/WordPress/WordPress/blob/master/wp-includes/js/dist/notices.min.js
- Used For: Reducing the bandwidth of network requests. WordPress will also try to combine JS into a single file unless you have the SCRIPT_DEBUG constant set to true.
- Identifying Features: .min.js extension. Lack of spaces and returns. eval() function.
- Learn More: https://wordpress.org/support/article/debugging-in-wordpress/
Minimized (Malware)
- Photo Credit: https://www.pokemon.com/us/pokedex/Tangela
- Code Example: Actual Malware.
- Used For: Breaking into your admin dashboard. Inserting spam links into your posts.
- Identifying Features: Code that is inserted inline into PHP and HTML files are usually not minimized. If you see an eval() like this in a .php file, especially if it is out of place or the same code in multiple files, it is usually malware. Use scanners.
- Learn More: https://sucuri.net/
jQuery
- Photo Credit: https://www.pokemon.com/us/pokedex/Baltoy
- Code is From: https://github.com/strangerstudios/paid-memberships-pro/blob/master/includes/profile.php
- Used For: jQuery made it easier to do DOM manipulation like this, toggling the display of fields based on other fields. Also made AJAX easier at the time.
- Identifying Features: jQuery or $, CSS selectors, .show(), .hide(), .ajax().
- Learn More: https://jquery.com/
AJAX
- Photo Credit: https://www.pokemon.com/us/pokedex/Claydol
- Code Example: https://github.com/bwawwp/bwawwp/blob/master/chapter-09/example-08.js
- Used For: Asynchronous JavaScript and XML. Asynchronous = making many calls back and forth with the web server once a page is loaded. Usually sending small amounts of data rather than the full page. Originally used XML as the primary data format. Now use JSON a lot.
- Identifying Features: ajaxurl, data, error, success, complete jQuery really made it easier to do AJAX requests. Before that you would use the XMLHttpRequest and use a bit more complicated code to manage the state of the requests. Modern JavaScript has the fetch command, which is more similar to how jQuery and other libraries use AJAX without requiring a library. (I’m looking for a good fetch example to add if you have one in some real WordPress code.)
- Learn More: https://codex.wordpress.org/AJAX_in_Plugins
JSON
- Photo Credit: https://www.pokemon.com/us/pokedex/Alakazam
- Code is From: https://github.com/WordPress/gutenberg/blob/master/packages/npm-package-json-lint-config/package.json
- Used For: Storing data in a way that is human readable and easy to parse. JSON can be pasted directly into JavaScript and interpreted. So the json_encode PHP function can be used to get variables into JS inline in a pinch. Using wp_localize_script is even better. JSON is perfect for sending data back and forth between JavaScript powered frontend and backend code. Also great for configuration files like those used for webpack and babel.
- Identifying Features: Curly brackets. Square brackets. Name-value pairs. .json, .config file extensions.
- Learn More: https://www.json.org/
Inline vs JS files
- Photo Credit: https://www.pokemon.com/us/pokedex/Rotom
- Code is From: https://github.com/strangerstudios/paid-memberships-pro/blob/master/includes/profile.php (Same example as the jQuery slide.)
- Used For: If you have a single use script meant to be used exactly on one page/template/etc, I still think it’s okay to put JavaScript into a <script> tag in your PHP code. In general tholugh, you should put JavaScript in a .js file and get data into your scripts through wp_localize_script and AJAX.
- Identifying Features: script tags. found in .php files. If one piece of inline JavaScript breaks, it will halt the execution of all inline JavaScript. One of the many reasons putting JS in its own file is better.
- Learn More: https://codex.wordpress.org/Using_Javascript
NPM
- Photo Credit: https://www.pokemon.com/us/pokedex/Sigilyph
- Code is From: https://github.com/strangerstudios/paid-memberships-pro/blob/dev/package.json
- Used For: Node Package Manager. It’s like the .org plugin repository, but for NodeJS modules.
- Identifying Features: package.json, package-lock.json, node_modules directory.
- Learn More: https://www.npmjs.com/, https://wordpress.org/gutenberg/handbook/designers-developers/developers/packages/packages-npm-package-json-lint-config/
ES5 vs ES6 vs ESNext
- Photo Credit: https://www.pokemon.com/us/pokedex/Porygon
- Code is From: https://gist.github.com/Shelob9/252011dff617b0fb9239865c6a045a37#file-4-js
- Used For: JavaScript is updated annually with new features. ES5 is the generally accepted baseline based on EcmaScript2015. ES6 added a lot of popular features. ESNext is shorthand for the latest. Use babel to polyfill your ESNext code.
- Identifying Features: asynx, fetch, await, promise
- Learn More: https://medium.freecodecamp.org/es5-to-esnext-heres-every-feature-added-to-javascript-since-2015-d0c255e13c6e
Babel
- Photo Credit: https://www.pokemon.com/us/pokedex/Ditto
- Code is From: https://github.com/strangerstudios/paid-memberships-pro/blob/dev/.babelrc
- Used For: Compiling ESNext/etc code for cross-browser support.
- Identifying Features: .babelrc file
- Learn More: https://babeljs.io/docs/en/
Webpack
- Photo Credit: https://www.pokemon.com/us/pokedex/Blastoise
- Code is From: https://github.com/WordPress/WordPress/blob/master/wp-includes/js/dist/notices.js
- Used For: Bundling dev code into build code. Monitors code changes to apply things like Babel to JS, compile SASS into CSS, and minify JS and CSS.
- Identifying Features: /******/ in js output. index.js, webpack.config.js
- Learn More: https://webpack.js.org/
REACT
- Photo Credit: https://www.pokemon.com/us/pokedex/Pikachu
- Code is From: https://github.com/CalderaWP/Caldera-Forms/blob/master/clients/privacy/components/FieldGroup.js
- Used For: “A JavaScript library for building user interfaces”. Started by Facebook. Library of “components” you can reuse across UI code. Manages “state” of UI to update things automatically as new data comes in. REACT Native for mobile development.
- Identifying Features: import { … } from ‘react’, class … extends React.Component, React.createElement, JSX (New way to use HTML inside of JavaScript)
- Learn More: https://reactjs.org/, https://webdevstudios.com/2019/01/03/headless-wordpress-with-react-and-nextjs-1/
Gutenberg Block
- Photo Credit: https://www.pokemon.com/us/pokedex/Crustle
- Code is From: https://github.com/ideadude/wp-minimal-block-example/blob/master/block.js
- Used For: The new block editor in WordPress (and elsewhere).
- Identifying Features: block.js, index.js, edit.js inside a /blocks/ or /blabla-block/ folder. window.wp.blocks, or wp.blocks
- Learn More: https://wordpress.org/gutenberg/handbook/designers-developers/developers/tutorials/block-tutorial/writing-your-first-block-type/